The unshift() method adds one or more values at the start of an array. If multiple values are passed in parameters, then all values are inserted at once at the beginning of the array, by the same order the parameters were passed.
Syntax
arr.unshift(value1[, ...[, valueN]])
Parameters
valueN: The values that need to be added in front of the array.
Example:
Response Body:
Test Script:
var resp = JSON.parse(responseBody);
//API Testing 'A Beginners View': JavaScript unshift() Method
accept_encoding = [];
var split = "gzip, deflate, br";
var split_value = split.split(",");
console.log("accept-encoding is: " + split_value);
accept_encoding.push(`${split_value[0]}`);
console.log(accept_encoding);
accept_encoding.push(`${split_value[1]}`);
console.log(accept_encoding);
accept_encoding.push(`${split_value[2]}`);
console.log(accept_encoding);
accept_encoding.unshift("Array First Element");
console.log(accept_encoding);
accept_encoding.unshift("Array Second Element");
console.log(accept_encoding);
accept_encoding.unshift("Array Third Element");
console.log(accept_encoding);
pm.environment.set("Unshift_Values", (accept_encoding));
Console Output:
In the above example, I used the previous post split() method (https://softwaretestingcafebyjency.blogspot.com/2020/10/JavaScript-split-Method.html) and continued to add the unshift() method to append more values at the start of the array. The accept_encoding.unshift()method is used to add values at the start of the array ‘accept_encoding’.
Important Notes:
Invoking unshift with n values all at once, or invoking it n different times with 1 value at a time will not yield the same results.
Let me explain with examples.
Snippet 1:
entityArr = [];
var split = "entities/1atqyUfo";
var split_value = split.split("/");
console.log("Splited values are: " + split_value);
entityArr.push(`${split_value[1]}`);
console.log(entityArr);
Console Output '1' will look as below:
Splited values are: entities,1atqyUfo
▶(1) ["1atqyUfo"]
Based on the above snippet, here the push() method will push the value of index 1 from the splitted string to array entityArr and output would be as ["1atqyUfo"] as seen in the output section.
To this, if I add the unshift() method as below:
Snippet 2:
entityArr = [];
var split = "entities/1atqyUfo";
var split_value = split.split("/");
console.log("Splited values are: " + split_value);
entityArr.push(`${split_value[1]}`);
entityArr.unshift("Array Begin");
console.log(entityArr);
Console Output now will be as below:
Splited values are: entities,1atqyUfo
▶(2) ["Array Begin", "1atqyUfo"]
Here the unshift() method will add the string ‘Array Begin’ to the start of the array as seen in the output section.
Again if I add multiple values all at once to the same array using unshift() method as below, look how my console output will change:
Snippet 3:
entityArr = [];
var split = "entities/1atqyUfo";
var split_value = split.split("/");
console.log("Splited values are: " + split_value);
entityArr.push(`${split_value[1]}`);
entityArr.unshift("Array Begin");
entityArr.unshift("Value1","Value2","Value3");
console.log(entityArr);
Console Output now will be as below:
Splited values are: entities,1atqyUfo
▶(5) ["Value1", "Value2", "Value3", "Array Begin", "1atqyUfo"]
Here the multiple values are added to the start of the array as we passed with unshift() method.
Now comes a twist, let’s pass few more values one by one again to the same array as below:
Snippet 4:
entityArr = [];
var split = "entities/1atqyUfo";
var split_value = split.split("/");
console.log("Splited values are: " + split_value);
entityArr.push(`${split_value[1]}`);
entityArr.unshift("Array Begin");
entityArr.unshift("Value1","Value2","Value3");
entityArr.unshift("Value4");
entityArr.unshift("Value5");
entityArr.unshift("Value6");
console.log(entityArr);
Console Output now will be as below:
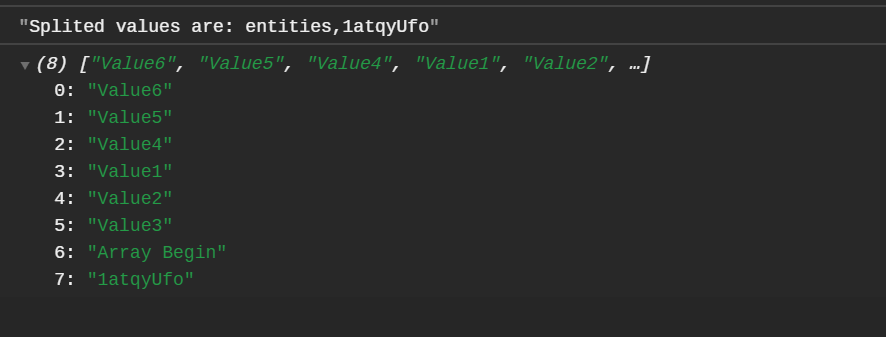
Value is inserted first come first shift basis.
Hope this clarified the unshift() method more clearly.
Happy Learning 😇